Starting Coding
When I started coding, I used Scratch, a very fun to use visual coding language. This helped my understand coding when I was only 5!
A few years later, I got into coding with more complicated languages, like HTML, JavaScript, and CSS.
I read library books about coding ALL the time, bought some, and just loved to code. With this tutorial I made, I hope you try to learn something like this too!
HTML Basics
HTML stands for HyperText Markup Language. It's the standard language used to create webpages. HTML tells the browser how to display content using elements written inside angle brackets like <tag>
.
The DOCTYPE Declaration
Every HTML document should begin with <!DOCTYPE html>
. This declaration tells the browser to render the page using HTML5 — the latest and most widely supported version of HTML.
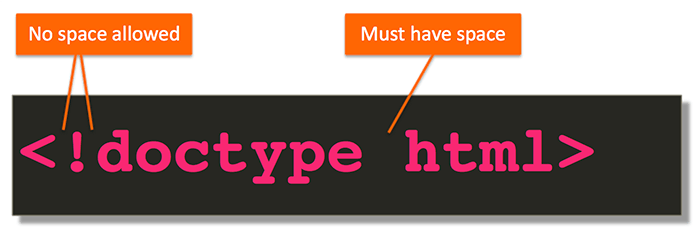
Without it, your webpage might not render correctly or consistently across different browsers. It's like the foundation of a house — you need it before building anything else.
The HTML Document Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Page Title</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>Hello, world!</h1>
<p>This is a paragraph.</p>
</body>
</html>
This is the basic layout of an HTML document. Let’s break it down:
The <head> Element
<title>
: Sets the text in the browser tab.<meta>
: Provides information like character encoding, author, and viewport settings for mobile devices.<link>
: Connects external files like CSS stylesheets.<script>
: Links or contains JavaScript code that adds interactivity to your page.
The <body> Element
This is where the visible content lives — the part users actually see and interact with:
- Headings:
<h1>
to<h6>
, with<h1>
being the biggest and most important. - Paragraphs:
<p>
for blocks of text. - Images:
<img>
with attributes likesrc
andalt
. - Links:
<a href="url">
to link pages or websites. - Lists:
<ul>
for unordered (bulleted) lists<ol>
for ordered (numbered) lists<li>
for list items inside either
- Tables:
<table>
,<tr>
,<td>
,<th>
to organize data in rows and columns. - Forms:
<form>
, used to collect user input with tags like<input>
,<textarea>
,<select>
, and<button>
.
Attributes
HTML elements often come with attributes that give extra information or functionality. For example:
<img src="image.jpg" alt="description">
<a href="https://example.com" target="_blank">Visit</a>
<input type="text" placeholder="Enter name">
Semantic HTML
Semantic tags clearly describe their meaning and purpose. They help with SEO, screen readers, and code organization. Examples include:
<header>
: Top of the page, often contains logo and navigation.<nav>
: Navigation links.<main>
: Main content area.<section>
: Groups related content.<article>
: Self-contained piece like a blog post.<footer>
: Bottom area, typically with copyright or contact info.
HTML is the structure — like the bones of your site. CSS is the style (clothes and colors), and JavaScript is the action (the muscles!).
When writing HTML, always close your tags, use nesting properly, and keep things organized. Valid HTML is easier to maintain, debug, and understand.
Learn More
Once you’re comfortable with the basics, check out topics like:
- HTML5 APIs (e.g., Geolocation, Local Storage)
- Embedding videos with
<video>
and audio with<audio>
- Creating accessible websites using ARIA labels and roles
The world of HTML is huge and powerful — and this is only a tiny bit!
CSS Basics (Cascading Style Sheets)
CSS helps you decorate your website and make it look really cool! It's like giving your HTML page a makeover with colors, spacing, and layout.
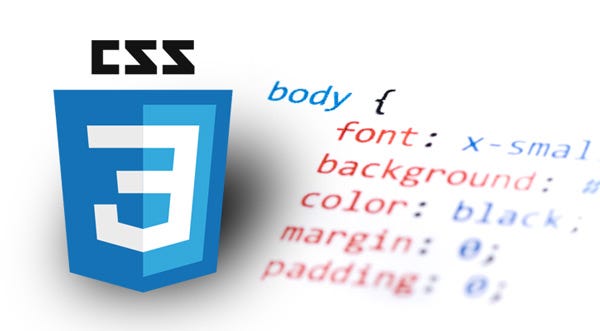
What Does CSS Look Like?
selector {
property: value;
}
A selector picks what HTML element you're styling. A property is what you want to change (like color), and the value is what you change it to.
Example:
body {
background-color: lightblue;
color: black;
}
This will make the page background light blue and the text color black!
Some Common CSS Properties:
color
: Changes the color of text.background-color
: Changes the background color of something.font-size
: Makes your text bigger or smaller.margin
: Adds space around things.padding
: Adds space inside the element.border
: Adds a border around boxes or text.
Ways to Use CSS:
- Inline CSS: You put the style right inside the HTML tag. (Not used a lot!)
- Internal CSS: You put the CSS in a
<style>
tag in the HTML<head>
. - External CSS: The best way! You link a separate file with all your CSS using
<link rel="stylesheet" href="style.css">
.
Making It Work on Phones and Tablets
With something called a "media query," you can make your site look good on all screen sizes!
@media (max-width: 600px) {
body {
font-size: 16px;
}
}
Cool Layout Tricks:
- Flexbox: Helps you line things up in a row or column.
- Grid: Helps you build a layout with rows and columns, like a table.
.container {
display: flex;
justify-content: center;
align-items: center;
}
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 20px;
}
Fun Extras:
- Transitions: Make things move smoothly.
- Animations: Let things move in cool ways.
- Variables: Let you reuse colors and values easily.
- Custom Fonts: You can use cool fonts from Google Fonts!
button {
transition: background 0.3s ease;
}
button:hover {
background: blue;
}
Tips for Writing CSS:
- Use classes instead of IDs most of the time.
- Keep things neat and add comments to organize your code.
- Use tools like the browser’s dev tools to test your CSS.
CSS makes your website colorful, stylish, and fun! Once you learn the basics, you can make any website look awesome and unique.
JavaScript Basics
JavaScript (or JS) is what makes your website do stuff. Like buttons that work, text that changes, or pop-ups that say “Hello!” It adds life to your website!
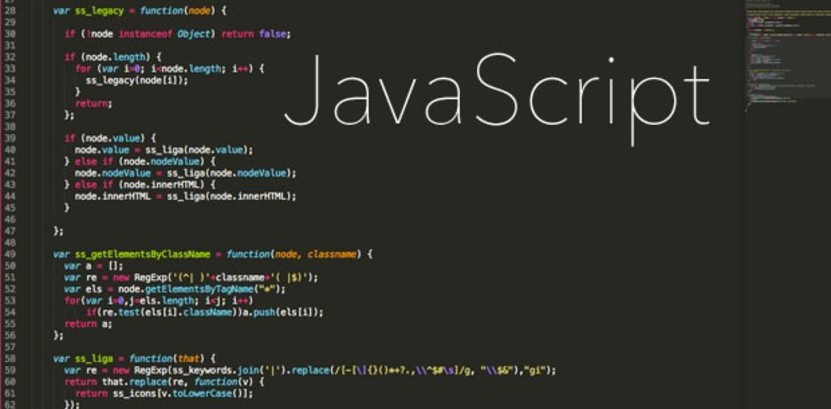
What Does JavaScript Look Like?
console.log("Hello World!");
🖥️ Fake Console Terminal
Common JavaScript Tools You’ll Use:
alert("Hi!")
: Shows a pop-up box with a message.console.log("Test")
: Prints messages to the console (for testing stuff).document.getElementById("demo").innerHTML = "Hi!";
: Changes text on the page!
Here’s a Cool Example:
// This changes the text in an HTML element with the ID "demo"
document.getElementById("demo").innerHTML = "JavaScript is awesome!";
Try it below!
Original Text
When Does JavaScript Run?
- When the page loads
- When you click something
- When you type or move the mouse
- Basically: It listens for events and then does cool stuff!
How Do You Add JavaScript?
- Inline: Inside HTML tags (but not recommended)
- Internal: Inside a
<script>
tag at the bottom of your HTML file - External: Link to a separate JS file like this:
<script src="script.js"></script>
What Can You Do With JavaScript?
- Make things show/hide when clicked
- Make games and cool effects
- Build interactive forms
- Fetch data from other websites using APIs
- Even build full apps with tools like React and Node.js
Tips for Learning JavaScript:
- Start small—play with buttons and text
- Use
console.log()
a LOT to see what’s happening - Break your code into steps
- Use websites like W3Schools, MDN, and JavaScript.info
JavaScript = making your website do things. Keep practicing, and soon you’ll be coding like a pro!
Example Scripts
Here you can find some cool scripts I made with HTML, CSS, and JavaScript!
<!DOCTYPE html>
<html lang="en">
<head>
<title>Page Title</title>
<link rel="stylesheet" href="back.css">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<a href="index.html" class="back-button">Back</a>
<h1>My First Heading</h1>
<p>My first paragraph.</p>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>My Simple Blog</title>
<link rel="stylesheet" href="example2.css">
<link rel="stylesheet" href="back.css">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<a href="index.html" class="back-button">Back</a>
<header>
<h1>My Blog</h1>
<nav>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
</header>
<main>
<article>
<h2>Blog Post Title</h2>
<p>Content of the first blog post...</p>
</article>
<article>
<h2>Another Blog Post</h2>
<p>Content of the second blog post...</p>
</article>
<!-- More articles can be added here -->
</main>
<footer>
<p>© 2025 My Simple Blog</p>
</footer>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>eCommerce Product Page</title>
<link rel="stylesheet" href="example3.css">
<link rel="stylesheet" href="back.css">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<a href="index.html" class="back-button">Back</a>
<header>
<div class="header-content">
<h1>Our Online Store</h1>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#new-arrivals">New Arrivals</a></li>
<li><a href="#best-sellers">Best Sellers</a></li>
<li><a href="#sale">Sale</a></li>
</ul>
<div class="cart-icon"></div>
</nav>
</div>
</header>
<main class="product-container">
<!-- Products-->
<section class="product" id="product1">
<img src="https://i.imgur.com/LXwNDDd.jpg" width="100%" alt="Product 1">
<h2>Product 1</h2>
<p class="price">$19.99</p>
<button class="add-to-cart">Add to Cart</button>
</section>
<section class="product" id="product2">
<img src="https://i.imgur.com/LXwNDDd.jpg" width="100%" alt="Product 2">
<h2>Product 2</h2>
<p class="price">$21.99</p>
<button class="add-to-cart">Add to Cart</button>
</section>
<section class="product" id="product3">
<img src="https://i.imgur.com/LXwNDDd.jpg" width="100%" alt="Product 3">
<h2>Product 3</h2>
<p class="price">$24.99</p>
<button class="add-to-cart">Add to Cart</button>
</section>
<section class="product" id="product4">
<img src="https://i.imgur.com/LXwNDDd.jpg" width="100%" alt="Product 4">
<h2>Product 4</h2>
<p class="price">$9.99</p>
<button class="add-to-cart">Add to Cart</button>
</section>
<!-- Additional eCommerce sections if needed -->
</main>
<footer>
<p>© 2025 Our Online Store. All rights reserved.</p>
</footer>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>Simple Survey Form</title>
<link rel="stylesheet" href="example4.css">
<link rel="stylesheet" href="back.css">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<a href="index.html" class="back-button">Back</a>
<main>
<h1>Feedback Survey Form</h1>
<form id="survey-form">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="age">Age:</label>
<input type="number" id="age" name="age" min="1" max="150">
<label for="feedback-type">Type of Feedback:</label>
<select id="feedback-type" name="feedback-type">
<option value="positive">Positive</option>
<option value="negative">Negative</option>
<option value="suggestion">Suggestion</option>
</select>
<fieldset>
<legend>Services Used:</legend>
<input type="checkbox" id="service1" name="services" value="service1">
<label for="service1">Service 1</label><br>
<input type="checkbox" id="service2" name="services" value="service2">
<label for="service2">Service 2</label><br>
<input type="checkbox" id="service3" name="services" value="service3">
<label for="service3">Service 3</label>
</fieldset>
<label for="comments">Additional Comments:</label>
<textarea id="comments" name="comments" rows="4"></textarea>
<button type="submit">Submit Feedback</button>
</form>
</main>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>Parallax Website</title>
<link rel="stylesheet" href="example5.css">
<link rel="stylesheet" href="back.css">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<a href="index.html" class="back-button">Back</a>
<div class="parallax-section" id="section1">
<h1>Welcome to Our Parallax Site</h1>
</div>
<div class="content-section">
<p>This is a regular content section. Scroll down to see the parallax effect.</p>
</div>
<div class="parallax-section" id="section2">
<h1>Immersive Experience</h1>
</div>
<div class="content-section">
<p>More content can go here. The next background will start with another scroll.</p>
</div>
<!-- Add more parallax and content sections as needed -->
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Digital Clock</title>
<link rel="stylesheet" href="back.css">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<style>
body {
font-family: 'Arial', sans-serif;
background-color: #000;
color: #00FF00;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
font-size: 3em;
}
</style>
</head>
<body>
<a href="index.html" class="back-button">Back</a>
<div id="clock">00:00:00</div>
<script>
function updateClock() {
const now = new Date();
let h = String(now.getHours()).padStart(2, '0');
let m = String(now.getMinutes()).padStart(2, '0');
let s = String(now.getSeconds()).padStart(2, '0');
document.getElementById("clock").textContent = `${h}:${m}:${s}`;
}
setInterval(updateClock, 1000);
updateClock(); // call immediately
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>To-Do List</title>
<link rel="stylesheet" href="back.css">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<style>
body {
font-family: Arial, sans-serif;
background: #f0f8ff;
padding: 30px;
}
h1 {
text-align: center;
color: #333;
}
input, button {
padding: 10px;
font-size: 16px;
}
ul {
list-style-type: none;
padding-left: 0;
}
li {
background: #dff0d8;
margin: 10px 0;
padding: 10px;
display: flex;
justify-content: space-between;
}
</style>
</head>
<body>
<a href="index.html" class="back-button">Back</a>
<h1>To-Do List</h1>
<input type="text" id="task" placeholder="Add a new task">
<button onclick="addTask()">Add</button>
<ul id="taskList"></ul>
<script>
function addTask() {
const taskInput = document.getElementById("task");
const taskText = taskInput.value.trim();
if (taskText === '') return;
const li = document.createElement("li");
li.innerHTML = `${taskText} <button onclick="this.parentElement.remove()">❌</button>`;
document.getElementById("taskList").appendChild(li);
taskInput.value = "";
}
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Typing Speed Tester</title>
<link rel="stylesheet" href="back.css">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<style>
body {
font-family: monospace;
padding: 30px;
background-color: #f5f5f5;
color: #333;
}
#test-text {
border: 1px solid #ccc;
padding: 10px;
margin-bottom: 10px;
}
#input-area {
width: 100%;
height: 100px;
}
#results {
margin-top: 20px;
font-weight: bold;
}
</style>
</head>
<body>
<a href="index.html" class="back-button">Back</a>
<h1>Typing Speed Tester</h1>
<p id="test-text">The quick brown fox jumps over the lazy dog.</p>
<textarea id="input-area" placeholder="Start typing here..." oninput="checkTyping()"></textarea>
<div id="results"></div>
<script>
const testText = document.getElementById("test-text").textContent;
let startTime;
function checkTyping() {
const input = document.getElementById("input-area").value;
if (!startTime) {
startTime = new Date();
}
if (input === testText) {
const endTime = new Date();
const timeTaken = (endTime - startTime) / 1000; // in seconds
const words = testText.split(" ").length;
const wpm = Math.round((words / timeTaken) * 60);
document.getElementById("results").textContent = `You typed at ${wpm} words per minute!`;
}
}
</script>
</body>
</html>
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Calculator</title>
<link rel="stylesheet" href="back.css">
<link rel="stylesheet" href="example9.css">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<a href="index.html" class="back-button">Back</a>
<div class="calculator-container">
<div class="calculator">
<input type="text" id="display" readonly>
<div class="row">
<button onclick="clearDisplay()">C</button>
<button onclick="appendOperator('/')">÷</button>
<button onclick="appendOperator('*')">×</button>
<button onclick="backspace()">⌫</button>
</div>
<div class="row">
<button onclick="appendNumber('7')">7</button>
<button onclick="appendNumber('8')">8</button>
<button onclick="appendNumber('9')">9</button>
<button onclick="appendOperator('-')">−</button>
</div>
<div class="row">
<button onclick="appendNumber('4')">4</button>
<button onclick="appendNumber('5')">5</button>
<button onclick="appendNumber('6')">6</button>
<button onclick="appendOperator('+')">+</button>
</div>
<div class="row">
<button onclick="appendNumber('1')">1</button>
<button onclick="appendNumber('2')">2</button>
<button onclick="appendNumber('3')">3</button>
<button onclick="calculate()" class="equals">=</button>
</div>
<div class="row">
<button onclick="appendNumber('0')" class="zero">0</button>
<button onclick="appendNumber('.')">.</button>
</div>
</div>
</div>
<script src="example9.js"></script>
</body>
</html>
CSS:
body {
background: #0e0f1a;
color: white;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
height: 100vh;
margin: 0;
display: flex;
justify-content: center;
align-items: center;
}
.calculator-container {
display: flex;
justify-content: center;
align-items: center;
width: 100%;
}
.calculator {
background: #1f1f2e;
padding: 20px;
border-radius: 20px;
box-shadow: 0 20px 50px rgba(0, 0, 0, 0.5);
width: 320px;
}
#display {
width: 100%;
height: 60px;
font-size: 28px;
text-align: right;
padding: 10px;
border: none;
border-radius: 10px;
margin-bottom: 15px;
background: #2c2c3d;
color: white;
box-sizing: border-box;
}
.row {
display: flex;
justify-content: space-between;
margin-bottom: 10px;
}
button {
flex: 1;
margin: 5px;
height: 60px;
font-size: 20px;
border: none;
border-radius: 10px;
background-color: #4e4e6a;
color: white;
cursor: pointer;
transition: background 0.2s;
}
button:hover {
background-color: #69698a;
}
.equals {
background-color: #ff914d;
color: black;
}
.equals:hover {
background-color: #ffa866;
}
.zero {
flex: 2;
}
JavaScript:
window.onload = () => {
const display = document.getElementById('display');
window.appendNumber = function(num) {
display.value += num;
};
window.appendOperator = function(op) {
const lastChar = display.value.slice(-1);
if ('+-*/'.includes(lastChar)) return;
if (display.value !== '') display.value += op;
};
window.clearDisplay = function() {
display.value = '';
};
window.calculate = function() {
try {
display.value = eval(display.value);
} catch {
display.value = 'Error';
}
};
window.backspace = function() {
display.value = display.value.slice(0, -1);
};
};
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Snake Game Extreme Cool Edition</title>
<link rel="stylesheet" href="example10.css">
<link rel="stylesheet" href="back.css">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<a href="index.html" class="back-button">Back</a>
<div id="game-container">
<canvas id="gameCanvas"></canvas>
<div id="countdown" class="hidden">3</div>
<button id="startButton">Start Game</button>
</div>
<script src="example10.js"></script>
</body>
</html>
CSS:
body {
background-color: #2f2f2f;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
color: white;
font-family: 'Arial', sans-serif;
}
#game-container {
position: relative;
}
#gameCanvas {
background-color: #000;
border: 2px solid #fff;
}
#startButton {
position: absolute;
bottom: 20px;
width: 100px;
height: 40px;
background-color: #4caf50;
color: white;
border: none;
font-size: 18px;
cursor: pointer;
border-radius: 5px;
}
#startButton:hover {
background-color: #45a049;
}
#countdown {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
font-size: 50px;
font-weight: bold;
color: #FF0000;
}
.hidden {
display: none;
}
JavaScript:
// Game Variables
const canvas = document.getElementById('gameCanvas');
const ctx = canvas.getContext('2d');
const grid = 20;
let snake, food, dx, dy, score, gameOver, countdownInterval, gameInterval;
canvas.width = 400;
canvas.height = 400;
const startButton = document.getElementById('startButton');
const countdownDisplay = document.getElementById('countdown');
// Start Button Click Event
startButton.addEventListener('click', startGame);
// Game Loop
function gameLoop() {
if (gameOver) return;
setTimeout(function() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawFood();
moveSnake();
drawSnake();
checkGameOver();
gameLoop();
}, 1000 / 15); // 15 frames per second
}
// Start New Game
function startGame() {
startButton.style.display = 'none'; // Hide the start button
countdownDisplay.classList.remove('hidden'); // Show countdown
score = 0;
dx = grid;
dy = 0;
gameOver = false;
resetGameState();
startCountdown(3);
}
// Countdown before the game starts
function startCountdown(seconds) {
let count = seconds;
countdownDisplay.textContent = count;
countdownInterval = setInterval(() => {
count--;
countdownDisplay.textContent = count;
if (count === 0) {
clearInterval(countdownInterval);
countdownDisplay.classList.add('hidden'); // Hide countdown
gameLoop(); // Start the game loop
}
}, 1000);
}
// Reset Game State
function resetGameState() {
snake = [
{ x: 9 * grid, y: 9 * grid },
{ x: 8 * grid, y: 9 * grid },
{ x: 7 * grid, y: 9 * grid }
];
food = generateFood();
}
// Snake Movement
function moveSnake() {
const head = { x: snake[0].x + dx, y: snake[0].y + dy };
snake.unshift(head);
if (head.x === food.x && head.y === food.y) {
score += 10;
food = generateFood();
} else {
snake.pop();
}
}
// Draw Snake
function drawSnake() {
snake.forEach(function(segment, index) {
ctx.fillStyle = index === 0 ? "#00FF00" : "#00CC00"; // Head in green
ctx.fillRect(segment.x, segment.y, grid, grid);
});
}
// Draw Food
function drawFood() {
ctx.fillStyle = "#FF0000";
ctx.fillRect(food.x, food.y, grid, grid);
}
// Generate Random Food Position
function generateFood() {
let foodX = Math.floor(Math.random() * (canvas.width / grid)) * grid;
let foodY = Math.floor(Math.random() * (canvas.height / grid)) * grid;
return { x: foodX, y: foodY };
}
// Check Game Over
function checkGameOver() {
if (snake[0].x < 0 || snake[0].x >= canvas.width || snake[0].y < 0 || snake[0].y >= canvas.height) {
endGame();
}
for (let i = 1; i < snake.length; i++) {
if (snake[0].x === snake[i].x && snake[0].y === snake[i].y) {
endGame();
}
}
}
// End Game
function endGame() {
gameOver = true;
alert(`Game Over! Score: ${score}`);
startButton.style.display = 'block'; // Show the start button again
}
// Control Snake Direction
document.addEventListener('keydown', function(event) {
if (event.key === 'ArrowUp' && dy === 0) {
dx = 0;
dy = -grid;
} else if (event.key === 'ArrowDown' && dy === 0) {
dx = 0;
dy = grid;
} else if (event.key === 'ArrowLeft' && dx === 0) {
dx = -grid;
dy = 0;
} else if (event.key === 'ArrowRight' && dx === 0) {
dx = grid;
dy = 0;
}
});
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Drag & Drop List Sorter</title>
<link rel="stylesheet" href="example11.css">
<link rel="stylesheet" href="back.css">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<a href="index.html" class="back-button">Back</a>
<div class="container">
<h2>Drag & Drop List Sorter</h2>
<ul id="sortable-list">
<li class="sortable-item" draggable="true">Item 1</li>
<li class="sortable-item" draggable="true">Item 2</li>
<li class="sortable-item" draggable="true">Item 3</li>
<li class="sortable-item" draggable="true">Item 4</li>
<li class="sortable-item" draggable="true">Item 5</li>
</ul>
</div>
<script src="example11.js"></script>
</body>
</html>
CSS:
body {
background-color: #f0f0f0;
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.container {
background-color: white;
padding: 20px;
box-shadow: 0 5px 20px rgba(0, 0, 0, 0.1);
border-radius: 8px;
text-align: center;
}
h2 {
margin-bottom: 20px;
}
#sortable-list {
list-style-type: none;
padding: 0;
}
.sortable-item {
padding: 15px;
background-color: #3498db;
color: white;
margin: 10px 0;
border-radius: 5px;
cursor: move;
transition: transform 0.2s ease;
}
.sortable-item:active {
transform: scale(1.05);
}
.sortable-item.dragging {
opacity: 0.5;
}
JavaScript:
const list = document.getElementById('sortable-list');
let draggedItem = null;
// When you start dragging an item
list.addEventListener('dragstart', (event) => {
draggedItem = event.target;
setTimeout(() => {
event.target.style.opacity = '0.5'; // Make it semi-transparent
}, 0);
});
// When you stop dragging an item
list.addEventListener('dragend', (event) => {
setTimeout(() => {
draggedItem.style.opacity = '1'; // Restore opacity after dragging
draggedItem = null;
}, 0);
});
// When a dragged item enters another list item
list.addEventListener('dragover', (event) => {
event.preventDefault(); // Allow dropping
});
// When the dragged item enters an item and it is over another list item
list.addEventListener('dragenter', (event) => {
if (event.target && event.target !== draggedItem && event.target.classList.contains('sortable-item')) {
event.target.style.border = '2px dashed #ccc'; // Add a visual cue
}
});
// When a dragged item leaves a list item
list.addEventListener('dragleave', (event) => {
if (event.target && event.target.classList.contains('sortable-item')) {
event.target.style.border = 'none'; // Remove the visual cue
}
});
// When the dragged item is dropped
list.addEventListener('drop', (event) => {
event.preventDefault();
if (event.target && event.target !== draggedItem && event.target.classList.contains('sortable-item')) {
event.target.style.border = 'none'; // Remove visual cue
const allItems = [...list.querySelectorAll('.sortable-item')];
const draggedIndex = allItems.indexOf(draggedItem);
const targetIndex = allItems.indexOf(event.target);
// Rearrange the list based on the positions of dragged and target items
if (draggedIndex < targetIndex) {
list.insertBefore(draggedItem, event.target.nextSibling);
} else {
list.insertBefore(draggedItem, event.target);
}
}
});